Scripting
The ADISRA SmartView software provides a powerful integration with c# scripts in multiple parts of the application.
C# is a programming language developed by Microsoft that runs on the .NET Framework. It is widely-used and known by developers and is a high-level language, it abstracts away most of the complex tasks and since it is a popular language, it will keep evolving and bringing more functionalities and improvements.
Why C# and not any other language?
The ADISRA SmartView was created to be a flexible and easy-to-use tool. With this in mind, the ADISRA SmartView was developed using C# since it is a language with such good performance which is vital for SCADA software and has so many great features. After making that decision, it was easy to choose C# as the scripting language. The users would be able to implement powerful code using a language that is greatly documented and supported by so many other developers.
Reference Description | URL |
Microsoft C# HomePage with Tutorials and Documentation | |
Microsoft C# Programming Guide | https://docs.microsoft.com/en-us/dotnet/csharp/programming-guide/ |
Great Documentation and Tutorial page with working examples | |
Another great page which includes a Tutorial, Tips and detailed explanation | |
Company leader in online education |
ADISRA SmartView Scripts
1. Overview
Let’s explore ADISRA SmartView Scripts now. They combine C# code with something else that makes them very powerful to connect the entire application.
The ADISRA SmartView is SCADA (Supervisory Control and Data Acquisition) software. ADISRA SmartView allows the users to manipulate all the data being collected or data being sent to different locations whether it is a Database, a PLC (Programmable Logic Controller) or any other device.
All the data must be stored in variables within ADISRA SmartView. The variables are called Tags and are available to be used in Scripts.
ADISRA SmartView was designed to connect devices, create amazing interfaces to display all the data. There is a large collection of Screen Objects created from the most basic elements like Labels, TextBoxes, ComboBoxes, CheckBoxes to more complex elements like MatrixGrids, MultiTagViewers, Charts, Trends, Alarms. The Scripts have access to those objects. So this means that with one single script you can add rotation to a Geometric Object using any Tags value, maybe add new items to a ComboBox, or add new Pens to a Trend object. The possibilities are endless.
It is very important to understand the architecture of the ADISRA SmartView and the scope of different modules before designing your application and writing the necessary scripts. The next section will explain the scripts found in different modules and their relationships, represented in the diagram below.
2. Scope and Relationship
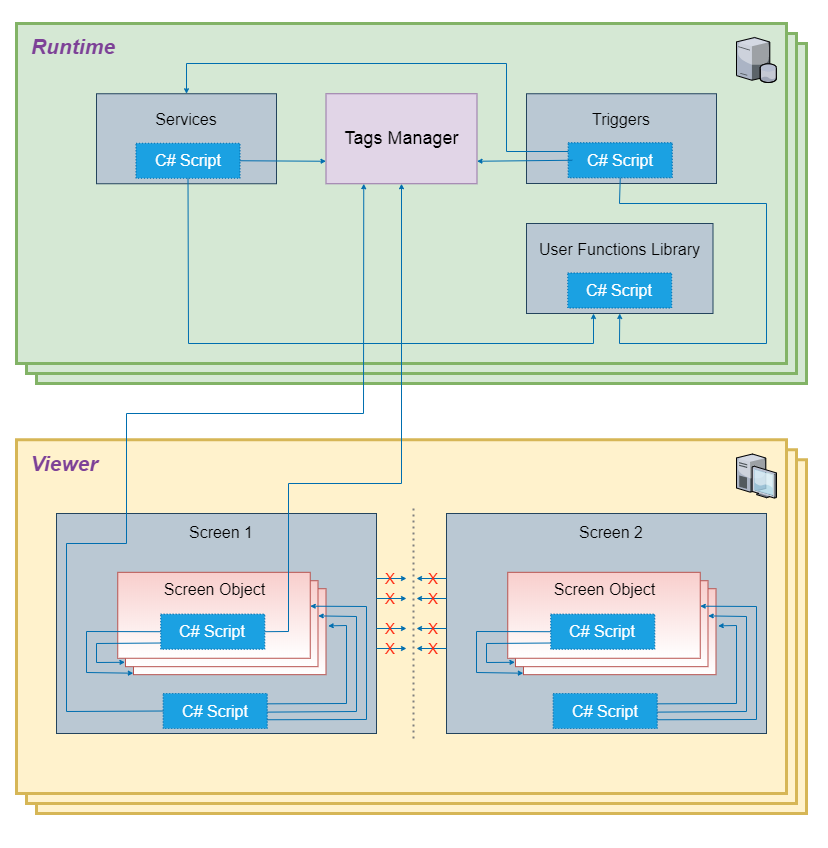
The above diagram shows the scope of the scripts and their relationship to other modules. All Graphics Scripts (Screen, Objects and Templates) are executed on the Viewer. The Services, Triggers and User Functions are executed on the Runtime. They all have access to the Tag Manager, which means that if a tag changes values, they will all be notified and will share the same tag value. The diagram also shows there is no relationship between 2 different screens. So a script from Screen 1 cannot be used to change a Screen Object’s property on Screen 2.
There are some particular cases where the script can be executed on the Runtime or the Viewer, it depends on the location of the Script. For example, the User Functions Library, if it is called from a Graphics Script, it will be executed in the Viewer but if it is called from a Service, it will be executed in the Runtime. It is also worth mentioning, that some System Functions Library are always executed in Runtime (i.e. SVTags and SVRecipe). As you can see, the Tags Manager is located in the Runtime, so whenever a Script needs the value of a Tag or needs to update a Tag, there will be a communication between Viewer and Runtime.
3. Script Locations
There are many different places a script can be written in the ADISRA SmartView. Please check the list below and pay attention to the comments.
Location | Execution | Allow Tags | Can change screen objects | Comments |
Screen Object Scripts | Viewer | Yes | Yes | The screen object scripts are executed in the Viewer. They can change other screen object’s properties on their scripts too, but they need to be in the same screen. If they call a System/User Function for example, it will be executed in the Viewer, unless it calls a System Function that changes a Tag (i.e. SVTags) or maybe a Recipe (i.e. SVRecipe). |
Screen Scripts | Viewer | Yes | Yes | Can only change screen objects located in the same screen. |
Services | Runtime | Yes | No | The Services are always executed in Runtime |
Triggers | Runtime | Yes | No | The triggers are always executed in Runtime. They can also execute Services. |
User Functions Library | Viewer/Runtime | No | No | Cannot change tags values or use them directly. But you can pass them as parameters and use its return values to change a tag. It can be executed in the Viewer or in Runtime, it depends which module is calling it. If a graphics is calling it (screen script or object script) it will execute in the Viewer. If a Service calls it, it will be executed in the Runtime. |
Template Object | Viewer | Yes | Yes | Any object added to the user object can change other object’s public properties inside the same template. The objects part of a Template cannot change objects outside this Template directly, but they can change tags that are configured to external objects. They can also have internal tags that can be used in Scripts. |
The following sections will introduce the features mentioned above and explain the scope of the Scripts while understanding the architecture.
4. Scripts and C#
The script, in the image below, identifies the C# language and the keywords and reserved words font color is changed.
It also compiles the code written every time it is saved (i.e. Services)
The red rectangle shows there is an error in the script. At the bottom of the image you can see the error in the engineering log.
The next image shows an example of a Screen Object Script. The same error was added to a button. This time the error is raised as soon as you remove the focus from the script, even before saving it.
Constants
The ADISRA SmartView scripts contain some constants that can also be used by the developers. Take a look at the table below with examples and descriptions.
Constant | Example | Description |
IsRunningWeb | if (IsWebRunning) | Checks if the current viewer is on the web or not. This constant is a bool type that returns true in case it is on the web viewer. |
5. Scripts and ScreenTags
The ADISRA SmartView allows you to create screen tags. The scope of those tags are only local to that screen and they can be used directly on the objects of the screen or in the Scripts. We will focus on Scripts in this section. The Screen Tags allow you to create a generic screen that can be used with different inputs, not restricting you to always use the same global tags.
They can be created in the Screen’s property grid as you can see in the image below.
Screen Tags can be used by adding the # token on the scripts. As soon as you type the # sign, all the screen tags will be listed in a drop-down menu to help you find them.
6. Scripts and Template Tags
The Template Objects work the same way as Screen Tags. Once a new template object is created, you will have the option to create Object Tags on the property grid that can also be accessed using the # token. This makes the template objects incredibly flexible and generic, allowing them to be used with different inputs.
7. Scripts and Tags
In ADISRA SmartView, all the Tags created in the application can be used in Scripts, simply type the “@” token as shown in the image below, and the Scripts will display all the Tags available.
In case the tag is a data type, the autocomplete will also help you find the inner tag like in the example below.
If the tag doesn’t exist, the ADISRA SmartView will allow you to create it by selecting its type and the document location, as shown in the image below.
Whenever a tag’s value is set in a Script, this tag change is sent to Runtime’s Tag Manager and it is responsible for notifying all Viewers.
8. Scripts and Screens
The Screens have 3 events where a Script can be written. To have access to those script windows you can either select Script View or Split View and select the Screen. Look for the Mode View component and make your choice.
The following image shows the script window in the Script View mode
As previously mentioned, Screens accept any C# compliant code and it also accepts tags with the @ token, User Functions, System Functions, and it can also manipulate Screen Object’s properties. The Screen Object properties will be explained in the next section in detail. These scripts accept multiple lines of code, but you cannot write functions nor classes. Think about it as a function already declared with no parameters or return value.
8.1 Screen Script Events
There are 3 types of events in Screen Scripts. OnOpen, OnWhile and OnClose.
OnOpen
Executes every time the current screen opens. It will be executed only once. It doesn’t matter if the screen is being opened by the Screen Object, part of the interface objects, or by the SVGraphics system function or any other way, this code will be executed once.
OnWhile
Executes multiple times while the screen is still opened. The frequency of its execution can be very low, around 300ms, and it depends on how much processing is happening on the Viewer. This is commonly used for animating the screen or checking for a specific condition, although you can use a trigger in case you expect certain conditions over a tag’s value.
OnClose
Executes every time the current screen is closed. It will be executed only once.
9. Scripts and Screen Objects
There are multiple Objects available in the ADISRA SmartView to be add to the screens. They fall under different categories like Geometric objects, Basic objects, Interface objects, Advanced objects and Charts. This document’s scope is to show all the properties and functions that can be used to manipulate those objects.
It is important to understand that those object properties and functions can only be used by Screen Scripts, the user must be in the Screen Script where the object was added, and used by Object Scripts that are on the same screen.
To have access to the properties and functions on the scripts, you will need the object name. By default, ADISRA SmartView already assigns a name to the objects when they are created, but the name can be changed if desired for better organization and maintenance.
9.1 Object Script Events
There are 6 common types of events in the Object Scripts. MouseUp, MouseDown, MouseWhile, MouseRightUp, MouseRightDown and MouseDoubleClick. For ComboBox object there is one more event called SelectionChanged. Let’s take a look at each event in further detail.
There are 2 ways to have access to the Object’s Script Events.
1) You can select the object, double-clicking on the object opens the Mode View, and change Mode View to Script View or select the Split View.
The following image shows the Rectangle’s Script Events in the Script View mode. Those same events are common to most of the objects
2) The second way to gain access to the script events using the Property Grid, after selecting the object as shown in the image below.
MouseUp
MouseUp is executed whenever the user releases the left button of the mouse over the selected object. This event is commonly used on actions that can be used on more critical actions and provides the user an opportunity yo quit the action. If the user selects a button, keeping the left mouse button depressed, then decides not to complete the action, the user should drag the mouse away from the selected button before he releases the left button, the event will not be executed.
MouseDown
MouseDown is executed whenever the user selected an object and depresses the left button of the mouse.
MouseWhile
MouseWhile is executed while the selected object is still clicked, between MouseDown and MouseUp events of the left button of the mouse.
MouseRightUp
MouseRightUp is executed whenever the user releases the right button of the mouse over the selected object.
MouseRightDown
MouseRightDown is executed whenever the user clicks down the selected object using the right button of the mouse.
MouseDoubleClick
MouseDoubleClick is executed when a double click is identified using the mouse left button.
SelectionChanged
SelectionChanged is executed whenever the selected item of a ComboBox changes.