How to Use the C# Language
Purpose of this article
This article aims to show information about the C# language, that is, what it is, how it works and how to use it in ADISRA SmartView.
What is the C# Language?
C# (pronounced “C sharp”) is a modern, general-purpose programming language developed by Microsoft. It was introduced in the early 2000s as part of the .NET framework and has since gained popularity for its versatility and performance.
In the C# language, there are several Conditional Structure that can be used to control the flow of program execution. The most used conditional structures are:
if, else, else if, switch-case, while, for and many others.
At the end of this article, we will also learn about ternary expressions, which can also be used in ADISRA SmartView.
Below we will learn how to use the main conditional structures in ADISRA SmartView.
“if” Conditional Structure
The conditional “if” structure is used to execute a block of code if a condition is true. It can be used alone or in conjunction with the else and else if structures.
Example:
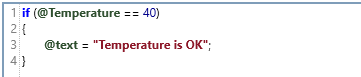
In the example above, if the value of the tag @Temperature is equal to 40, the text “Temperature is OK” will be assigned to the tag @text. If condition is not true, the code block will not be executed.
“else” Conditional Structure
The conditional structure “else” is used together with the “if” and allows executing a block of code if the condition of the “if” is false.
Example 01:
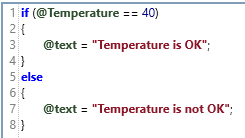
In the example above, if the value of the @Temperature tag is equal to 40, the text “Temperature is OK” will be assigned to the @text tag. If false, the text “Temperature is not OK” will be assigned to the @text tag.
Example 02:
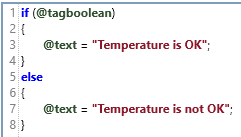
In the example above, if the value of the @tagboolean tag is true, the text “Temperature is OK” will be assigned to the @text tag. If the condition is not true, the text “Temperature is not OK” will be assigned to the @text tag.
Example 03:
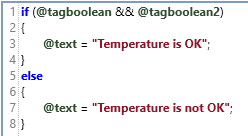
In the example above, if the value of the @tagboolean “AND” @tagbollean2 tag is true, the text “Temperature is OK” will be assigned to the @text tag. If the condition is not true, the text “Temperature is not OK” will be assigned to the @text tag.
Example 04:
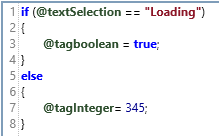
In the example above, if the value of the tag @textSelection is equal to the text “Loading”, the value “true” will be assigned to the tag @tagboolean. If not, the @tagInteger tag will be assigned the value 345.
“else if” Conditional Structure
The “else if” conditional structure is used when there are several conditions to be checked in sequence. It is used in conjunction with “if” and “else”.
Example 01:
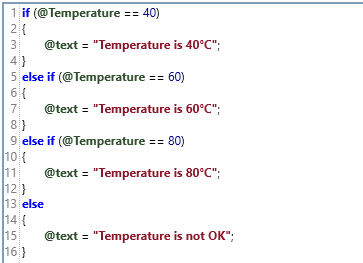
Note: There are no limits on the number of conditions in this structure. In the example above we used 4 conditions, but you can use 50 conditions if necessary.
Note²: In each condition, we use the equals operator (==) to compare the @Temperature tag with a certain value. In addition to this operator, it is possible to use other logical operators. To learn more about logical operators, see the article “How to Use Logical Operators in C#“.
Note³: Be careful when using the operators (=) and (==). The operator (=) is used to assign values to a tag. And the operator (==) is used for comparison between values and tags. Therefore, the comparison operator (==) is used inside the condition and the assignment operator is used inside the block.
“Switch-Case” Conditional Structure
The “Switch-Case” conditional structure Checks multiple conditions and executes different blocks of code based on the values of an expression.
Example:
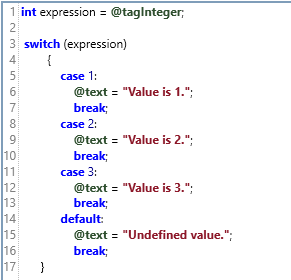
In the example above, after executing the script, the value of the tag @tagInteger will be assigned to the variable “expression”. The Conditional Switch-case structure will check if the variable ‘expression” is equal to each case. If the variable “expression” is equal to 1, the text “Value is 1.” will be assigned. If it is not 1, the next case will run and so on.
The “break” function in a switch-case structure in C# is used to stop the execution of the current block of code and exit the switch-case structure. When a case is matched and its block of code is executed, execution continues for subsequent cases unless a break is encountered. If you don’t use the “break” function, execution would continue for subsequent cases even if the matching case was found.
The “default” function in a switch-case structure in C# is used to define a block of code that will be executed if none of the conditions of the previous cases are met. In other words, the block of code inside the “default” is executed when the tested value does not match any of the specified cases.
Note: According to the example above, it is not possible to add a tag inside the Switch-Case conditional structure. So, in the example above, it was necessary to assign the value of the @tagInteger tag to a local variable called “expression” and then use that variable in the switch-case.
“While” Conditional Structure
The “while” Conditional Structure is used to execute a block of code repeatedly as long as a condition is true.
Example:
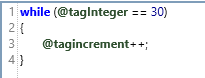
Note: Be careful when using this conditional structure, as the script will run every 300ms. This may affect the performance of the application.
“For” Conditional Structure
The “for” Conditional Structure is a controlled loop with a counter. It lets you specify an initialization, a continuation condition, and an incrementing expression.
Example:
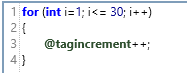
The “For” conditional structure consists of three parts:
Initialization: int i = 1; – In this part, a variable called i is initialized with the value 1. It is executed only once, at the beginning of the loop.
Condition: i <= 5 – The condition is checked before each iteration of the loop. As long as the condition is true, the code block inside the loop is executed. In this case, the loop will run as long as the value of i is less than or equal to 5.
Increment: i++ – After each iteration of the loop, the increment part is executed. In this case, we increment the value of i by 1 at each iteration.
So the @tagIncrement tag will be incremented 30 times.
Ternary Expression (If – Else)
The ternary operator is a resource for making decisions with a similar objective to that of if/else but which is encoded in only one line.
Ternary operator syntax:
boolean expression ? code 1 : code 2
If the boolean expression returns true, the code declared right after the question mark (?) is executed; otherwise, the system will execute the code following the colon (:).
The main differences between the conditional if/else structure and the ternary operator is that the latter, as already mentioned, is described in just one line of code, executing just one statement, and is usually contained in a larger statement.
The ternary expression allows you to add an if/else condition to object properties.
See the example below:
We need to show in a textbox object if the temperature is ok or not. So, I can do it in two ways using If/Else conditional structure.
1- Creating a Conditional If/Else structure as shown at the beginning of this article. Therefore, I will need to put the code below running in a triggers or services document or on the screen’s OnWhile.
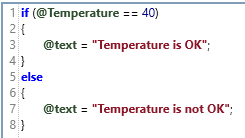
2- Or, we can directly add a ternary expression inside the “Text” property of the textbox object.
@text = (@Temperature == 40) ? “Temperature is OK” : “Temperature is not OK”;
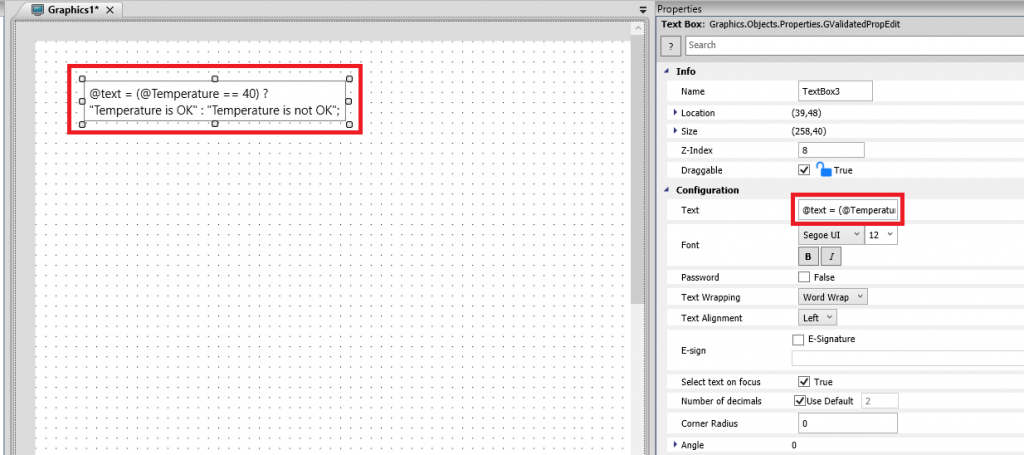